Bookkeeping Automation Transformation
How we helped a mid-sized service company reduce bookkeeping time by 78% and increase data accuracy to 99.8% through automation of bank transactions, OCR invoice processing, and Python scripting
Client Overview
A mid-sized IT consulting firm with 120+ employees handling over 5,000 monthly transactions across multiple bank accounts and clients.
Our client was experiencing rapid growth, with the number of clients more than doubling in two years. Their financial operations were struggling to keep pace with this expansion, as the finance team was spending excessive time on manual bookkeeping tasks. With monthly transaction volumes exceeding 5,000 entries across multiple bank accounts, credit cards, and client billing systems, the three-person accounting team was spending nearly 80% of their time on data entry rather than financial analysis and strategic decision support.
5,000+
Monthly transactions requiring manual processing
800+
Monthly vendor invoices requiring manual entry
120+
Hours spent monthly on manual bookkeeping tasks
Key Challenges
-
Manual Bank Transaction Processing
Time-consuming manual reconciliation of multiple accounts
-
Invoice Processing Bottlenecks
Tedious manual entry of vendor invoice details
-
Repetitive Data Entry
Accounting staff performing the same tasks repeatedly
-
Error-Prone Processes
Manual data entry leading to accuracy issues
The Challenge
The client's rapid growth had created overwhelming manual bookkeeping processes that were error-prone, time-consuming, and limiting the finance team's strategic contributions.
The client was facing several critical challenges with their bookkeeping operations:
- The finance team was spending approximately 120 hours each month manually processing bank statement transactions from 6 different business accounts
- Processing more than 800 vendor invoices monthly required manual data entry, with each invoice taking an average of 4-5 minutes to process
- Recurring transactions like monthly subscriptions, payroll entries, and standard accruals were being re-entered each month without any automation
- The manual processes resulted in a 6% error rate, requiring additional time for correction and verification
- Month-end close was taking 15-18 days due to the extensive manual reconciliation and data entry requirements
- The finance team had limited capacity for financial analysis and strategic support due to administrative burden
Additionally, the manual nature of their bookkeeping processes was making it difficult to scale operations to accommodate their growing business. With plans to expand services and potentially open new office locations, they needed a more efficient and scalable approach to financial record-keeping.
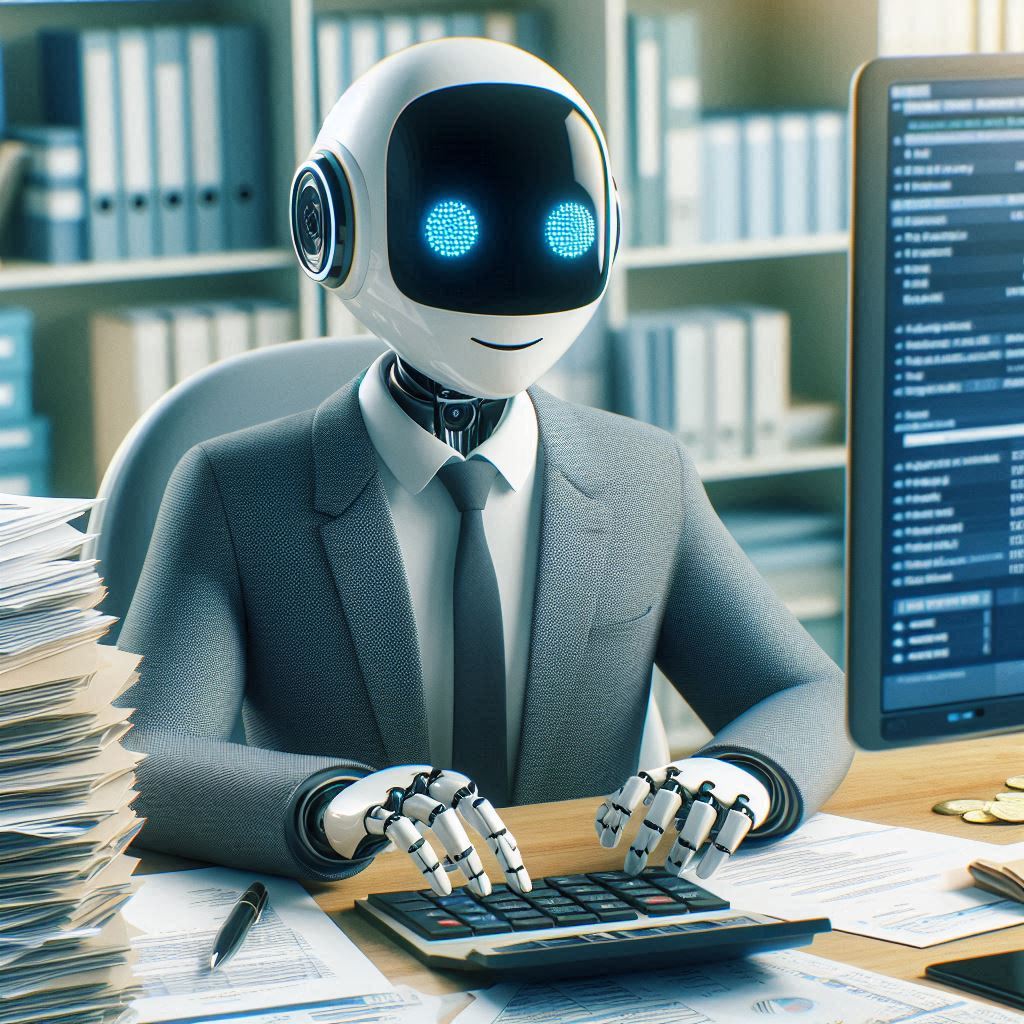
6%
Manual Entry Error Rate
15-18 Days
Month-End Close Time
Bookkeeping Efficiency Assessment
Our Approach
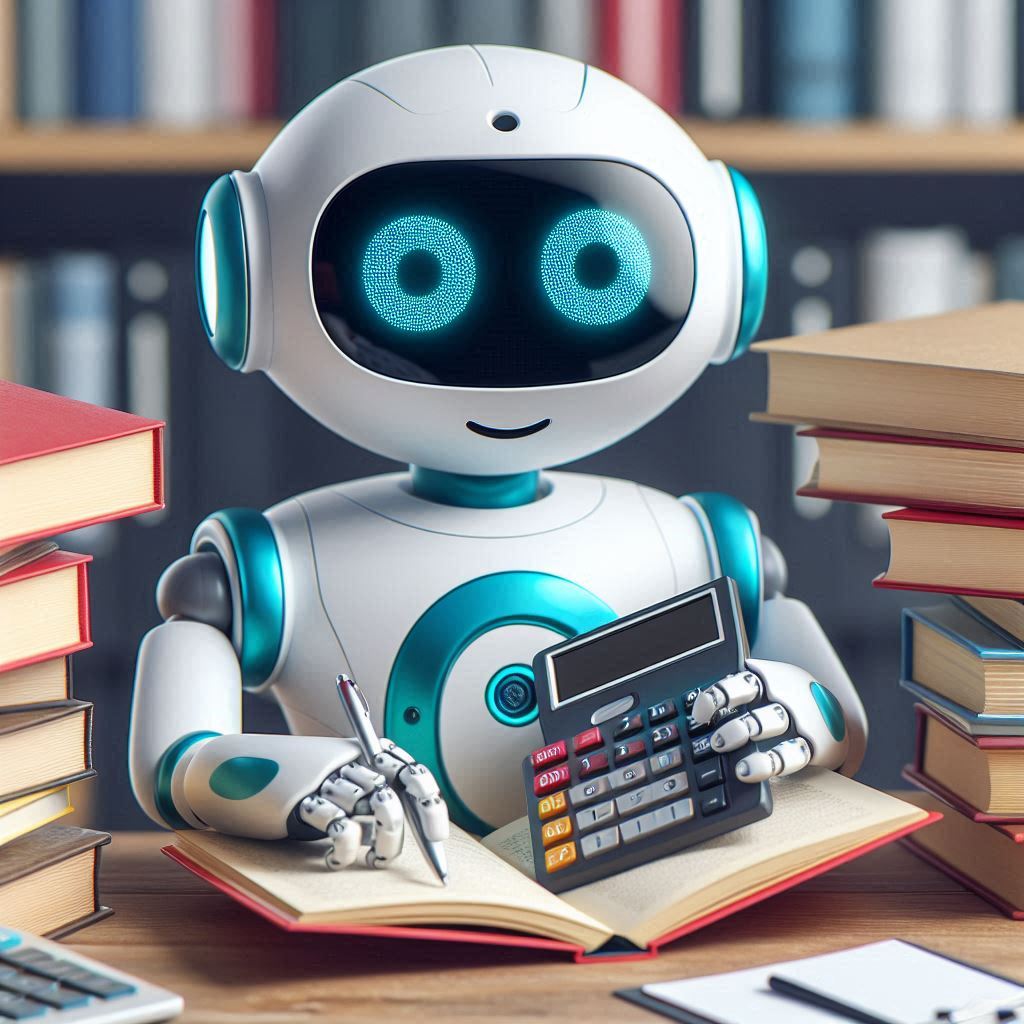
Process Optimization Assessment
We conducted a thorough analysis of the client's current bookkeeping processes:
- Documented current workflows and identified key bottlenecks
- Analyzed transaction patterns across all bank accounts
- Categorized invoices by type, frequency, and processing requirements
- Mapped recurring bookkeeping tasks for automation potential
- Evaluated existing accounting software capabilities and integration options
Automation Strategy Development
Based on our assessment, we created a comprehensive automation strategy:
- Designed bank feed automation with intelligent transaction categorization
- Developed OCR-based invoice processing workflow with validation rules
- Created Python automation scripts for repetitive bookkeeping tasks
- Established data validation and exception handling protocols
- Designed a phased implementation approach to minimize disruption
Technology Implementation
We implemented the automation solutions with focus on integration and reliability:
- Set up secure API connections with banking systems
- Implemented and trained OCR invoice processing system
- Developed and tested Python scripts for recurring transactions
- Created custom dashboard for monitoring automation performance
- Established exception management workflow for unusual transactions
Training & Transition Management
We ensured successful adoption of the new automated systems:
- Developed comprehensive training program for the finance team
- Created detailed documentation and standard operating procedures
- Implemented parallel processing during transition period
- Established metrics to monitor automation performance
- Provided ongoing support for refinement and optimization
The Solution
Bank Statement Automation
We developed an automated system for processing bank transactions that eliminated manual data entry and improved categorization accuracy.
Key Features:
- Secure API integration with all banking institutions
- Machine learning-based transaction categorization
- Pattern recognition for recurring transactions
- Automated matching with existing vendor profiles
- Exception flagging for unusual transactions
- Daily reconciliation with accounting system
OCR Invoice Processing
We implemented an advanced OCR system that automatically extracts relevant data from invoices and enters it into the accounting system.
Key Features:
- Advanced OCR technology with 99.8% accuracy
- Automatic field recognition (amount, date, vendor, etc.)
- Multi-format support (PDF, image, email, etc.)
- Validation rules to catch potential errors
- Automated approval workflow integration
- Automatic filing and document management
Python Automation Scripts
We developed custom Python scripts to automate repetitive bookkeeping tasks and complex calculations.
Key Features:
- Automated monthly journal entries for recurring items
- Prepaid expense amortization calculations
- Revenue recognition schedule automation
- Inter-company transaction reconciliation
- Custom financial report generation
- Data validation and error checking routines
Technical Implementation Details
Bank Transaction Automation
# Python code for bank transaction processing
import pandas as pd
from sklearn.ensemble import RandomForestClassifier
from bank_api_connector import BankConnector
def process_transactions(bank_id, start_date, end_date):
# Connect to bank API and fetch transactions
connector = BankConnector(bank_id)
transactions = connector.get_transactions(start_date, end_date)
# Convert to DataFrame for processing
df = pd.DataFrame(transactions)
# Apply ML categorization model
categories = transaction_classifier.predict(df['description'])
df['category'] = categories
# Match with existing vendors
df['vendor_id'] = df['description'].apply(match_vendor)
# Prepare for accounting system import
accounting_entries = prepare_journal_entries(df)
# Push to accounting system
return accounting_system.batch_create_entries(accounting_entries)
OCR Invoice Processing
# Python code for OCR invoice processing
import cv2
import pytesseract
import re
from invoice_processor import InvoiceParser
def process_invoice(file_path):
# Read the invoice image/PDF
image = cv2.imread(file_path)
# Preprocess the image for better OCR
processed_img = preprocess_image(image)
# Extract text using OCR
text = pytesseract.image_to_string(processed_img)
# Parse invoice details using specialized parser
parser = InvoiceParser()
invoice_data = parser.parse(text)
# Validate extracted data
validation_errors = validate_invoice_data(invoice_data)
if validation_errors:
return {'status': 'review', 'errors': validation_errors}
# Create invoice in accounting system
return accounting_system.create_invoice(invoice_data)
Recurring Transaction Automation
# Python code for automated recurring transactions
from datetime import datetime
import calendar
import pandas as pd
from accounting_api import AccountingSystem
def generate_monthly_entries():
# Connect to accounting system
accounting = AccountingSystem()
# Get current month details
now = datetime.now()
last_day = calendar.monthrange(now.year, now.month)[1]
# Load recurring transaction templates
templates = pd.read_csv('recurring_transactions.csv')
# Generate entries for each template
entries = []
for _, template in templates.iterrows():
entry = {
'date': f"{now.year}-{now.month:02d}-{template['day']:02d}",
'account_debit': template['account_debit'],
'account_credit': template['account_credit'],
'amount': calculate_amount(template),
'description': template['description'].format(month=now.strftime('%B')),
'reference': f"{template['reference']}-{now.strftime('%Y%m')}"
}
entries.append(entry)
# Create journal entries in accounting system
results = accounting.batch_create_journal_entries(entries)
# Log results and return summary
log_results(results)
return summarize_results(results)
Results & Impact
Key Performance Improvements
78%
Reduction in Bookkeeping Time
99.8%
Data Entry Accuracy
5 Days
New Month-End Close Time
₹18L
Annual Cost Savings
Business Impact
The automated bookkeeping solution delivered transformative results across the organization:
Massive Time Savings
The finance team reduced time spent on transaction processing from 120 hours to just 26 hours per month. Bank transaction processing time decreased by 85%, and invoice processing time was reduced by 74%. This freed up significant capacity for higher-value activities.
Improved Financial Insights
With the finance team spending less time on data entry, they increased their focus on financial analysis by 320%. Management now receives financial insights weekly instead of monthly, with customized dashboards providing real-time visibility into key metrics.
Enhanced Accuracy and Compliance
Data entry errors decreased from 6% to just 0.2%, significantly reducing the need for corrections. The automated system enforces consistent classification of transactions, improving tax compliance and financial reporting reliability.
Scalable Foundation for Growth
The company can now handle 3x their current transaction volume without adding accounting staff. This has supported their expansion into two new markets without any corresponding increase in bookkeeping costs.
ROI Analysis
Investment Category | Cost | Annual Benefit | ROI | Payback Period |
---|---|---|---|---|
Bank Transaction Automation | ₹4,50,000 | ₹7,80,000 | 173% | 6.9 months |
OCR Invoice Processing | ₹3,85,000 | ₹6,40,000 | 166% | 7.2 months |
Python Automation Scripts | ₹2,20,000 | ₹4,10,000 | 186% | 6.4 months |
Training & Implementation | ₹1,85,000 | Not directly quantifiable | N/A | N/A |
Total Project | ₹12,40,000 | ₹18,30,000 | 148% | 8.1 months |
Automation Performance Dashboard
As part of the solution, we implemented a real-time dashboard for monitoring automation performance and identifying areas for further optimization.
Transaction Processing Performance
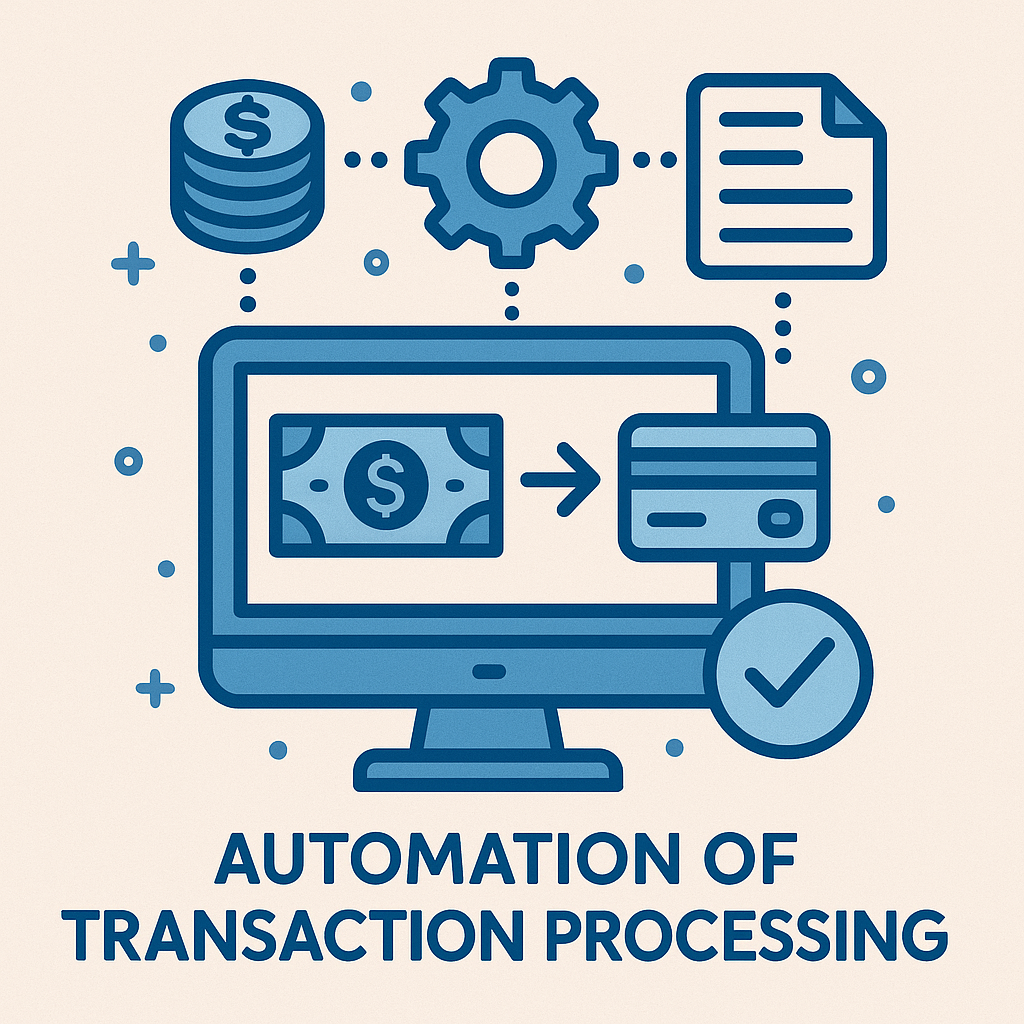
OCR Processing Accuracy
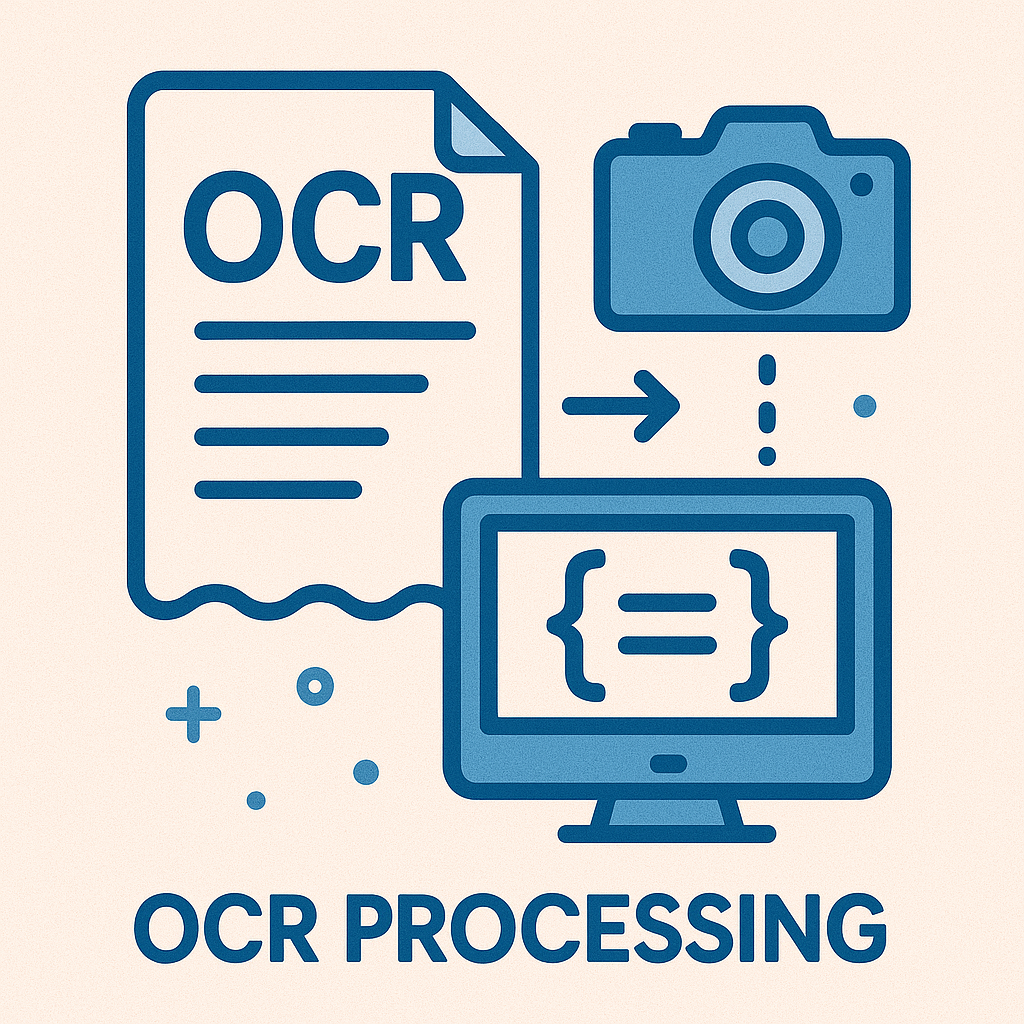
Key Performance Metrics
Metric | Before Automation | After Automation | Improvement | Status |
---|---|---|---|---|
Daily Bank Transaction Processing Time | 3.5 hours | 32 minutes | 85% reduction | Excellent |
Invoice Processing Time (per invoice) | 4-5 minutes | 45-60 seconds | 80% reduction | Excellent |
Month-End Close Duration | 15-18 days | 5 days | 70% reduction | Excellent |
Data Entry Error Rate | 6.0% | 0.2% | 97% reduction | Excellent |
Finance Team Capacity for Analysis | 15% | 63% | 320% increase | Excellent |
Transaction Processing Capacity | 6,000/month | 18,000/month | 200% increase | Excellent |
The bookkeeping automation solution that Sompalli & Co implemented has been transformative for our finance team. What once took up 80% of our time is now largely automated, allowing us to focus on providing valuable financial insights to our management team. The combination of bank feed automation, OCR invoice processing, and Python scripts for repetitive tasks has eliminated the most tedious aspects of our work while significantly improving accuracy. We've reduced our month-end close time from over two weeks to just 5 days, and the finance team now has capacity to support our growth strategy rather than just keeping up with transaction processing.
Implementation Timeline
Phase 1: Assessment
Week 1-2
- Process analysis
- Requirements gathering
- Solution design
Phase 2: Bank Integration
Week 3-5
- Bank API integration
- Transaction categorization
- Testing & refinement
Phase 3: OCR Implementation
Week 6-8
- OCR system setup
- Template training
- Validation rules creation
Phase 4: Python Automation
Week 9-10
- Script development
- Testing & validation
- Scheduling system setup
Phase 5: Training & Handover
Week 11-12
- Team training
- Documentation
- Support transition
Transform Your Bookkeeping Processes
Let's discuss how our automation solutions can free your finance team from manual data entry and unlock their potential for strategic analysis.